Unicode has enabled the internet as we know it! It has largely resolved the issues with Mojibake (garbled text) between different character sets being used.
Unicode is essentially a huge dictionary with mappings (for example, U+058E) that point to symbols we can use (֎). In the past, there were issues when different countries, corporations, or individuals all had different ideas about which character mapping should correspond to which symbols.
This means you'd often try to read webpages ��like�� thisⅇ↫⋀₿≝┿⣶⤚⺬⨺➄⧻⒏⥱➯⢃⢻⽱⓽⅘⋵⟖➝
❐≷↼Ⲝ┃ℵ
⸺⧷ⱟℱ⥹⧖⏗ₓ⡐⟄
┸⓳⡷⊢⠒┣⋰⡫.
Messy, right?
Unicode compliance now allows us to not only reliably use different languages other than English, but it also enables us to use other unique characters, like Egyptian hieroglyphics (𓀀 𓂕 𓁎), Sumerian Cuneiform characters (𒀖 𒁝𒃿), or emojis (
).
You can look at every emoji defined in the Unicode character set here.
Using emojis has been proven to boost engagement by 25.4% in certain situations, which explains why — based on 6.7 billion tweets over the last decade — emoji use has never been higher.
But just knowing that Unicode has enabled consistent emoji use isn't enough - there are still plenty of questions around including emojis, such as:
- How can we create reusable React components for them?
- How can we ensure the emojis are accessible for screen readers?
- What are the best practices when using them?
- Should we be using the emojis themselves or use the mapping instead?
Let's dig in! 
Emoji usage in React
There are multiple ways to include emojis in a codebase, but some are better than others.
Some of your options are:
- To copy and paste the emoji inline:

- To reference the Unicode identifier/mapping of the emoji HTML entity, like:
& #x1F603
; - To copy and paste the emoji inline, then wrap it in a HTML element:

- Install a dependency to deal with it
All of these would work! But "making it work" isn't always the optimal solution, so let's discuss the benefits and drawbacks to each of these approaches.
Using emojis inline
This is probably the simplest solution.
Copy and paste the emoji, and then the job's done.
In addition, screen readers are smart enough to know how to read emojis. So, simply doing this would leave your application utilizing emoji, and, for the most part, they'd be accessible.
But emoji often aren't as plain as screen readers can describe. They often take on a second meaning.
For example, do you know these "second meanings" of emojis?



The goat emoji is an acronym for the Greatest Of All Time, so people may often say something like the greatest rapper of all time (GOAT) is Tupac, or the greatest hockey player of all time (GOAT) is Wayne Gretzky.
The snake emoji is commonly used to describe people backstabbing, or being two-faced.
The clapping hands are commonly used to emphasize a point, like
this
between
each
word. Note that this particular type of writing is terrible for screen readers and discouraged for keeping your content friendly to screen readers. If you are interested in reading more about accessible emoji usage, here is an excellent resource, and so is this one.
But screen readers can't convey that meaning. Even if they did, with language changing so much, the definition could be wrong and would need to constantly be updated.
It's for this reason I would advise against using emoji inline.
That's one emoji approach ticked off. Let's talk about another.
Use the HTML entity Unicode mapping
You can use emojis hex or decimal code points from Unicode directly in your code, so that something like this:
<!DOCTYPE html> <div> <h1>Unicode Character</h1> <h3 style="display: inline">(U+1F436)</h3> <h3 style="display: inline">Dog Face</h3> <h3 style="display: inline">🐶</h3> </div>
Would render the below:
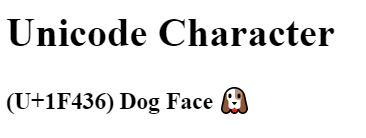
You could find these hex/decimal representations pretty easily, too. Here is an example of a huge list of emoji HTML hex codes.
Or you can find them via a lookup table available here.
Even using this method still doesn't fix the issues we mentioned about second meanings being lost, and, as a developer, I think it is harder to work with.
It's much easier to see the emojis in your code like this: 😃
, rather than read their mappings like so: & #x1F603
;
When you copy/paste an emoji directly, you immediately know what the emoji is and the context in which it is used. Emojis keep their semantic meaning a little better, and I'd argue that they are simpler to work with.
Wrap the inline emoji in a DOM Element
This is the best approach. You can simply wrap an inline emoji with a basic DOM element, something like this:
<span role="img" aria-label="dog">🐕</span>
This allows you to add better alt text if you think what a screen reader would pick is unclear with your writing.
If you are unsure what a screen reader would read for each emoji, you can search on Emojipedia, and the title of the emoji is what a screen reader would likely say.
Some examples are:
: Smiling face with hearts
: Globe showing Asia-Australia
: Person in suit levitating
Writing quality alt text is hard, and if you decide to re-word what the default text is slightly to better convey your meaning, remember that emotion matters.
For optimal reuse, you could make a very simple functional component that passes in all the necessary meta-data, something like this:
import React from 'react'; const Emoji = props => ( <span className="emoji" role="img" aria-label={props.label ? props.label : ""} aria-hidden={props.label ? "false" : "true"} > {props.symbol} </span> ); export default Emoji;
Then this component could be imported and used in a standardized way throughout the codebase:
<Emoji symbol="🐑" label="sheep"/>
With this method, you ensure a consistent and reusable pattern to use emoji in your codebase, and because <span>
displays inline by default, it can be used right in the middle of text with minimal CSS rework.
You can provide sensible defaults for the components properties, such as defaulting the aria-hidden
to false
if that also suits your use cases.
Doing so ensures your emojis are accessible, where you can explain any second meanings or extra explanation you want to accompany your emoji.
Install a dependency to deal with it
Installing a dependency makes the job easier, but it is generally less configurable if you need to do something specific or as a unique use case just for you.
There are several great npm packages available: emoji-picker-react, Unicode Emoji, or node-emoji. They are all easily and similarly installed.
For example, emoji-picker-react has a really simple setup. To include it in your dependencies, simply run npm i emoji-picker-react
. It offers a dropdown option for the emoji you want to choose.
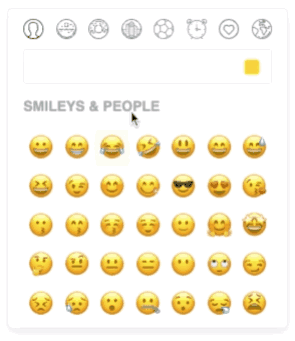
An emoji dropdown selector found here.
The npm package does use React Hooks, so you will need to use React 16.8 or higher!
The docs have a useful explanation of how to include it also:
import React, { useState } from 'react'; import Picker from 'emoji-picker-react'; const App = () => { const [chosenEmoji, setChosenEmoji] = useState(null); const onEmojiClick = (event, emojiObject) => { setChosenEmoji(emojiObject); }; return ( <div> {chosenEmoji ? ( <span>You chose: {chosenEmoji.emoji}</span> ) : ( <span>No emoji Chosen</span> )} <Picker onEmojiClick={onEmojiClick} /> </div> ); };
Conclusion
I hope this has been informative! 
There are multiple ways to add emojis into your React app, but with accessibility and reusability in mind, a simple functional component should fit almost every single use case.
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.